使用角度的正弦和餘弦函數,將長方形的寬度和高度乘以正確的係數,以獲得旋轉後的角點座標。
下面為一個將一個長方形的四個點作45度旋轉的簡單範例:
import cv2
import numpy as np
import math
base_x = 100
base_y = 100
width = 100
height = 50
angle = 45
# 計算長方形中心點座標
center_x = base_x + (width // 2)
center_y = base_y + (height // 2)
# 將角度轉換為弧度
angle_rad = math.radians(angle)
# 計算長方形四個角的相對座標
cos_val = math.cos(angle_rad)
sin_val = math.sin(angle_rad)
x = width / 2
y = height / 2
# 計算四個角點座標
point1 = (int(center_x - x * cos_val + y * sin_val), int(center_y - x * sin_val - y * cos_val))
point2 = (int(center_x + x * cos_val + y * sin_val), int(center_y + x * sin_val - y * cos_val))
point3 = (int(center_x + x * cos_val - y * sin_val), int(center_y + x * sin_val + y * cos_val))
point4 = (int(center_x - x * cos_val - y * sin_val), int(center_y - x * sin_val + y * cos_val))
print("Point 1:", point1)
print("Point 2:", point2)
print("Point 3:", point3)
print("Point 4:", point4)
# 創建空白影像
image = np.zeros((500, 500, 3), dtype=np.uint8)
# 轉換座標為Numpy陣列
pts = np.array([point1, point2, point3, point4], dtype=np.int32)
# 繪製多邊形
cv2.polylines(image, [pts], True, (0, 255, 0), thickness=2)
# 顯示結果
cv2.imshow('Rectangle', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
顯示結果如下
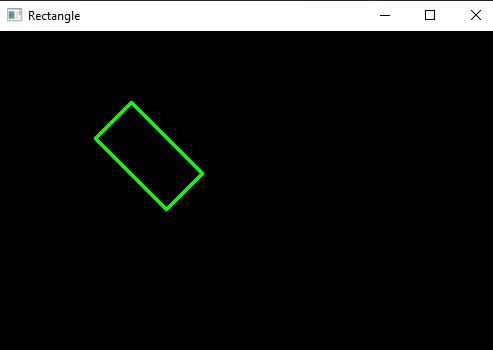